According to Citrusbug, 1.3 million websites use React to design their appearance and UI. Moreover, over 200,000 websites use React in the USA alone. Hence, this goes to show that hiring React developers has changed the way businesses build user interfaces with a component based architecture.
Moreover, one of the core concepts in React is the use of props, which can pass data between components. However, as applications grow in complexity, ensuring that components receive the correct type of props becomes crucial.
Therefore, PropTypes provides a mechanism to validate the types of props passed to components. Hence, this helps developers catch bugs and maintain codebases.
So, in this guide, we’ll look into what PropTypes are and how to use them effectively. Moreover, we will look at the challenges present and some best practices.
What are PropTypes?
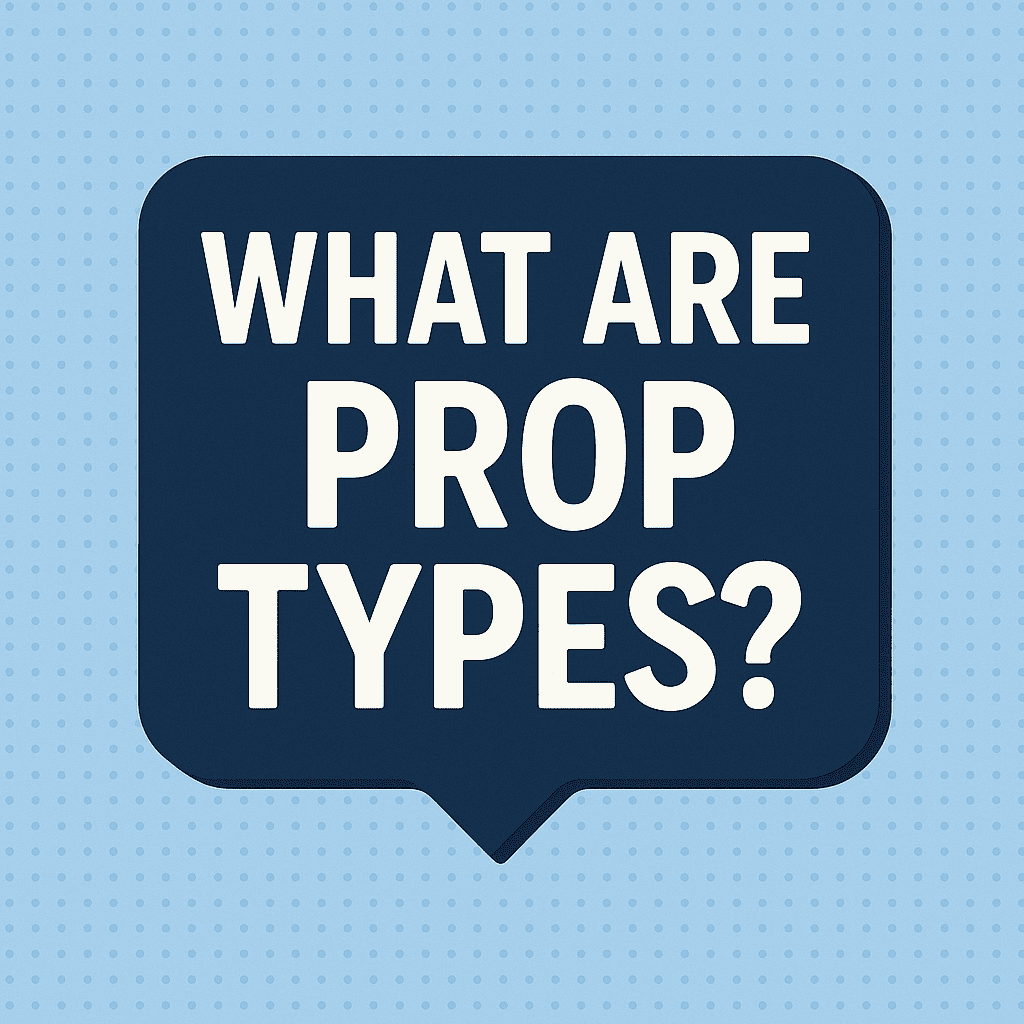
PropTypes in React are a way to validate the data types of props that pass into components. Moreover, you can think of them as a set of rules that define what kind of data your component should receive. Also, when you build a user interface, you can pass incorrect data types or forget data values.
React PropTypes also serve as a safety measure by informing you in the console of your browser while you’re developing if you supply data that doesn’t fit expectations.
Additionally, they don’t prevent your app from operating, but they can help you identify flaws early and warn you about any problems. Hence, this is useful in large applications where you can reuse components in many places.
Types of PropTypes
- Basic Types
The basic types are the regular data types developers use in TypeScript. It includes numbers and strings. Furthermore, it also includes objects and arrays. Also, you can use them by attaching the PropTypes method.
- Multiple Types
PropTypes also allows developers to set the type of values your props should be and the expected value. Moreover, it allows developers to set a limited set of values or multiple data types for a prop. Furthermore, you can do this by using PropTypes.oneof() and PropTypes.oneofType () validators.
- Custom Types
You know how to validate props with default validators. But you can also create your own validators. Let’s say you wish to confirm that a prop’s value is what you anticipate. Therefore, establishing a custom validator is one way to accomplish this. Creating a method that accepts three arguments, props, propName, and componentName, is the first step in creating a custom validator.
Why Use PropTypes?
PropTypes gives your React components an extra layer of type safety without requiring you to switch to a whole type system like TypeScript. Moreover, they help developers quickly identify what props they need. Hence, this serves both as a development tool and as living documentation. Moreover, this can be especially useful when onboarding new developers to a project or maintaining code over time.
Additionally, PropTypes also makes debugging easier. When something goes wrong with a component, the console warnings provided by PropTypes can give you clues about what’s missing or incorrectly passed. Therefore, instead of hunting through multiple files or guessing what a component needs, PropTypes offers immediate feedback that can save hours of debugging time.
Usage of PropTypes
Developers can import the PropTypes package into their component code before using a specific static property to specify the intended data types of props. For instance, you may specify that the name must be a string and that the age may be an optional integer if a component needs both a name and an age.
This type of definition acts like a contract. If a developer tries to use the component and forgets to pass the name or passes a number instead of a string, React will notify them in the console during development.
This prevents bugs that could cause parts of your UI to behave unexpectedly. Moreover, what’s especially useful is that PropTypes covers a wide range of data types. Also, you can validate simple types like strings and numbers. Furthermore, you can validate even complex ones like arrays and functions. Moreover, there’s also support for nested structures and enumerated values. Hence, this allows you to describe almost any shape of data a component might require.
How to Install and Import PropTypes?
- Install PropTypes with a Package Manager
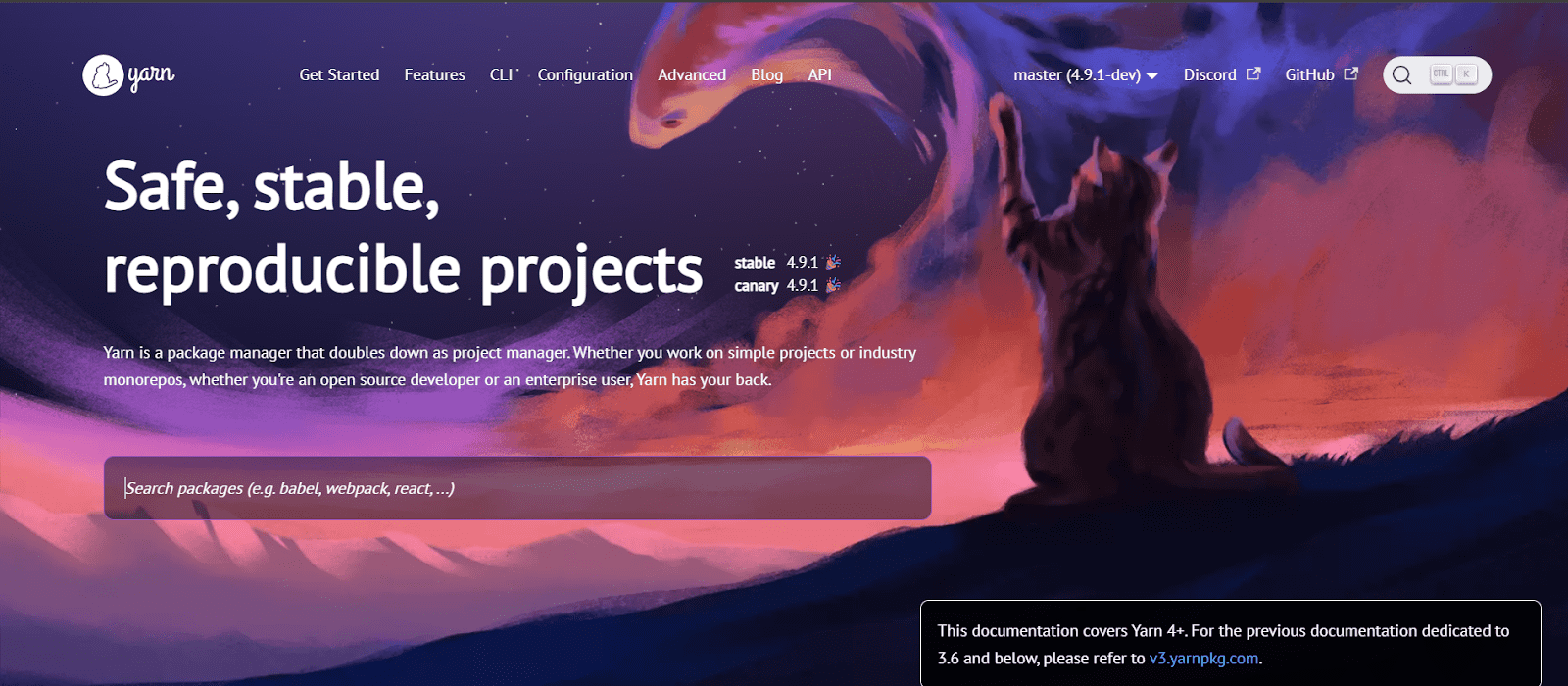
PropTypes may be added to your project using a JavaScript package management tool such as Yarn or npm. Additionally, these tools are in charge of overseeing your project’s dependencies and external libraries. Also, installing PropTypes is a one time setup process. This is due to the fact that the functionality is made available across your program after installation.
This phase guarantees that all users of the codebase have access to the same validation capabilities, whether you’re working in a team or starting a project from scratch.
- Import PropTypes into a Component
Importing PropTypes into any file where you intend to utilize them comes next after the package has been installed. Additionally, this is done at the beginning of the code, often next to your import declarations for React. Additionally, you may declare the anticipated prop types that your components will receive and import PropTypes, which provides you with access to a range of validators.
This is a crucial step since React won’t recognize the type checking rules you set if PropTypes aren’t imported. The import opens the PropTypes utility methods, which allow you to define what kind of data each prop should take, whether it is a simple string or a more complex object.
- Define PropTypes
After importing, you should define PropTypes for your components. This is often done by adding a special property to the component. This is where you assign a list of expected prop keys and their respective data types. Moreover, this declaration acts like a set of instructions for how the component should be used. Other developers will thus understand precisely what each prop implies and what kind of data to expect.
Furthermore, when the application is in development mode, React will display a warning message in the browser console if a prop is missing or if you give the wrong type. These warnings are quite useful for quickly detecting incorrect use and fixing issues early in the development cycle.
- Don’t use PropTypes in Production
You should use PropTypes only in development environments. So, when you build your application for production, React automatically strips out these checks to optimize performance. This means your end users won’t experience any slowdown or extra load caused by prop validation. However, you still get the full benefits of type safety during development.
Challenges of React PropTypes
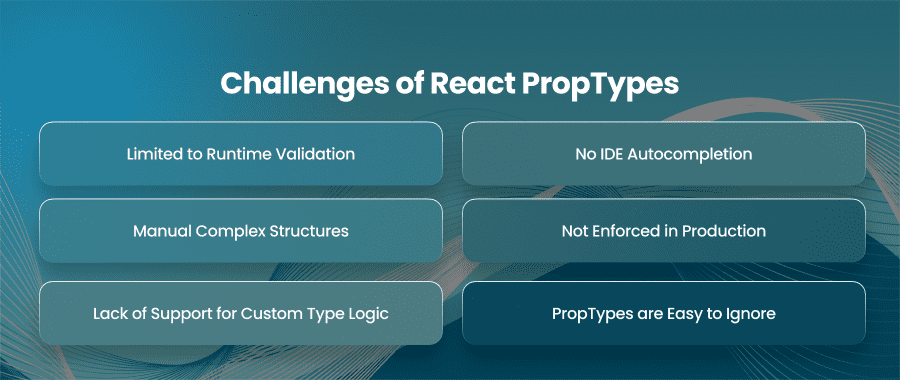
Limited to Runtime Validation
PropTypes only work during runtime. Hence, this means that the type checks and warnings occur while your application is running in development mode, not during the build or completion phase. As a result, any type of issues won’t be caught until the component is actually rendered. Therefore, this can delay the discovery of bugs, especially in larger applications where not every component is always active during testing.
No IDE Autocompletion
Another downside of using PropTypes is the lack of integration with modern development tools. So, when you use TypeScript, your editor provides helpful features like auto completion and immediate syntax feedback. Moreover, these features improve the developer experience and reduce cognitive load.
As PropTypes are a run time solution, they don’t offer this level of tooling support. Moreover, you won’t get any real time suggestions or validations in your IDE as you type, which can slow down development and make it easier to overlook small errors.
Manual Complex Structures
While PropTypes can handle arrays and nested shapes, defining complex or deeply nested data structures can become tedious. For example, if a component has a prop that is an array of objects, it could be required to construct multiple layers of nested definitions for PropTypes. This increases the likelihood of mistakes, but it also makes the code harder to understand and update.
Not Enforced in Production
PropTypes do not enforce prop validation in production builds. This makes sense from a performance standpoint, as it avoids adding extra processing to the end user experience. However, it also means that any issues that go past development validation will not be flagged in production. This can lead to bugs or broken UI behavior in live environments. To make sure nothing falls between the cracks, developers must be extra careful in their testing and development procedures.
Lack of Support for Custom Type Logic
PropTypes offer a set of predefined validators, like string and array. While it does allow for some custom validators, the process is less intuitive and error prone. So, writing and reusing custom validation logic requires a deep understanding of how PropTypes works under the hood. Hence, this adds complexity when trying to enforce business rules or non standard prop formats. So, this often leads teams to look for more flexible alternatives.
PropTypes are Easy to Ignore
Since PropTypes only throw warnings and not actual errors, they are easy to overlook and ignore. Moreover, the warnings appear in the console, but they don’t stop your app from functioning. Hence, this can result in a situation where invalid props persist in a codebase, thus creating inconsistencies and making the application harder to debug later.
Best Practices for Using PropTypes
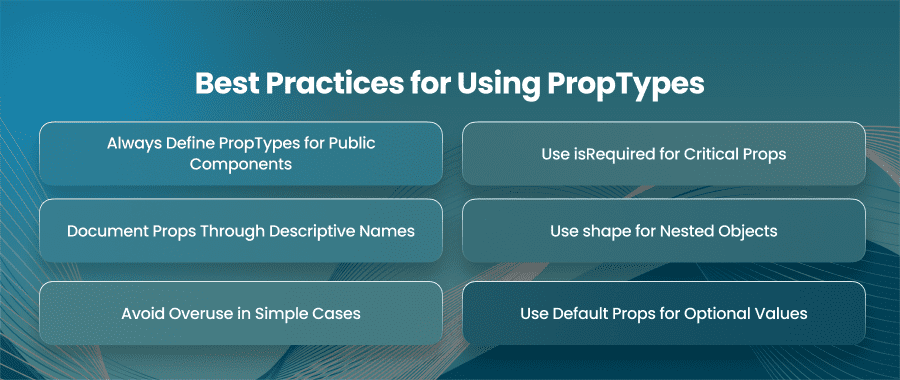
Always Define PropTypes for Public Components
Any component that is used across different parts of your application, especially those that are shared or reusable, should have clearly defined PropTypes. Moreover, this acts as a contract between the component and its consumers, making it clear what props are expected and what their types should be. So, when other developers use the component, these type definitions reduce confusion and ensure consistent behavior.
Use isRequired for Critical Props
React PropTypes offer an .isRequired modifier to indicate that a particular prop must be provided. You should use this feature for props that are necessary for the component to function properly.
For instance, if a prop controls core logic or is required for rendering key UI elements, marking it as required ensures that missing it will trigger a visible warning during development. Also, this can prevent a range of bugs that result from undefined values or missing data.
Document Props Through Descriptive Names
Since PropTypes act as documentation for your components, prop names need to be understandable and instructive. This is a great technique that greatly improves the readability and maintainability of your code, even if it’s not technically necessary. For example, when someone sees userEmail, it’s immediately more meaningful than a vague name like emailprop.
Use shape for Nested Objects
When dealing with props that are objects with multiple properties, it’s better to use the shape validator. This allows you to describe the internal structure of the object. So, this defines exactly what properties are expected and their types. Moreover, this practice is especially useful for props like user or config that bundle multiple related values.
Furthermore, providing structure at this level helps ensure the component receives all the required data in the correct format and reduces the chance of runtime errors caused by missing or incorrectly typed properties.
Avoid Overuse in Simple Cases
PropTypes are useful, but there is no need to go overboard with overly detailed validations for simple and internal use components. For example, if a component is small and you only use it within a single parent component, then a verbose PropTypes can introduce unnecessary clutter.
Use Default Props for Optional Values
React enables you to define default values for props using defaultProps. This is especially useful when a prop is optional, but your component logic depends on a fallback value. For example, a button component can have an option label prop with a default value. Moreover, this approach ensures your component behaves predictably even when some props are omitted.
Final Words
Validating component attributes during construction is simple and efficient using React PropTypes. Their simplicity and ease of use make them an essential tool for preserving code quality and enhancing teamwork in React projects, even though they are not as good as TypeScript.