Error handling is an essential aspect of writing strong software, particularly in Go, where error handling is treated differently compared to other mainstream programming languages like Java or Python.
Basic Concepts
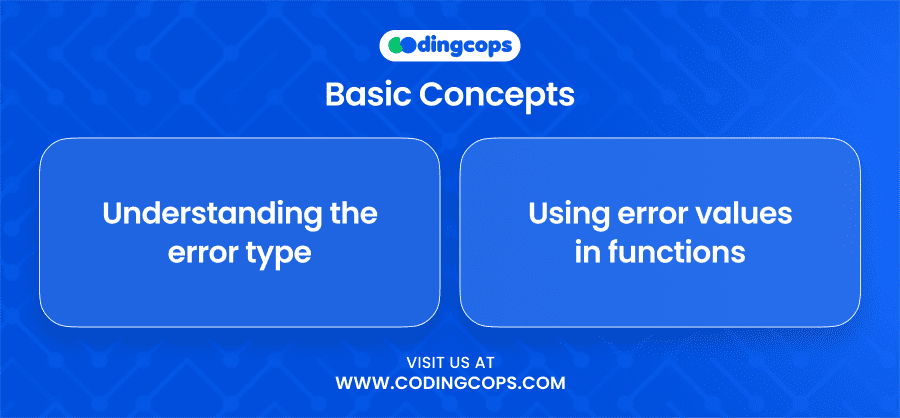
Understanding the Error Type
In Go, an error is an interface type defined in the built-in ‘error’ package. This interface requires any type implementing it to have a method ‘Error() string’. This simplicity allows for easy integration of custom error types in your applications.
Errors in Go provide a way to convey what went wrong without the complexities of exception handling found in other languages.
Using Error Values in Functions
Typically, Go functions that can encounter problems return an error as their last return value. If a function executes successfully without any issues, the error is nil; otherwise, it holds an appropriate error object describing what went wrong. This approach encourages checking errors where they occur and handling them appropriately.
Error Wrapping and Unwrapping
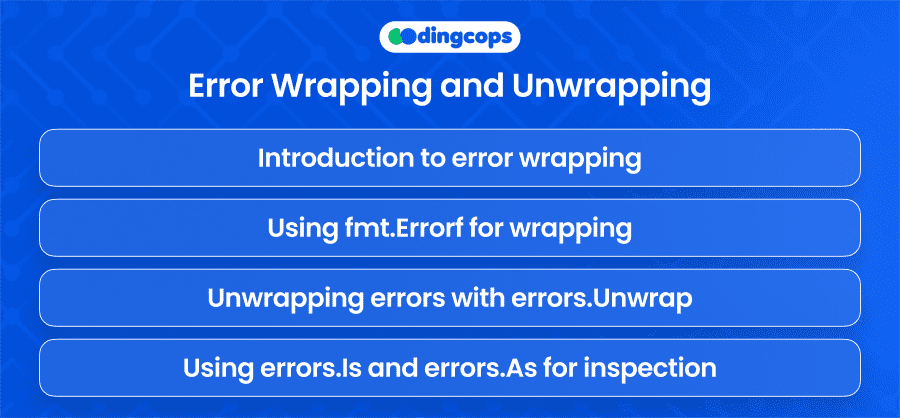
Introduction to Error Wrapping
Error wrapping in Go is a technique used to add additional context to an error without losing the original error information. This is important for debugging, as it allows GO developers to track issues through the layers of application logic.
Using fmt.Errorf for Wrapping
The ‘fmt.Errorf’ function in Go 1.13+ supports error wrapping directly using the ‘%w’ verb. When an error is wrapped using ‘fmt.Errorf’, you can add additional context that will help during debugging while preserving the underlying error.
Unwrapping errors with errors.Unwrap
The standard library’s ‘errors’ package provides the ‘Unwrap’ function, which returns the result of calling the ‘Unwrap’ method on the error, if available. This is useful when you need to examine the original error after multiple layers of wrapping.
Using errors.Is and errors.As for Inspection
‘errors.Is’ and ‘errors.As’ are helpful for checking and asserting error types:
- ‘errors.Is’ checks if any error in the chain of wrapped errors matches a specific error.
- ‘errors.As’ finds the first error in the chain that matches a specific error type and provides access to it.
Best Practices
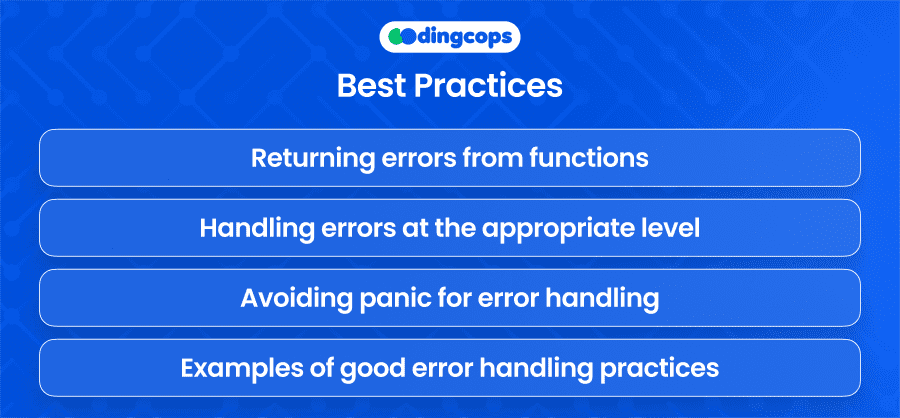
- Returning Errors from Functions
It’s best practice to return the error as the last value in your function’s return signature when something might go wrong. This standard approach helps maintain consistency and readability in your codebase.
- Handling Errors at the Appropriate Level
Avoid the temptation to handle errors immediately as they occur unless necessary. Sometimes, it’s better to return errors to higher layers of your application where you have more context or the ability to take more appropriate actions.
- Avoiding Panic for Error Handling
Reserve ‘panic’ for truly exceptional situations that are not part of the normal operation of your program. For regular error handling, use error values and checks.
Examples of Good Error Handling Practices
Logging Errors
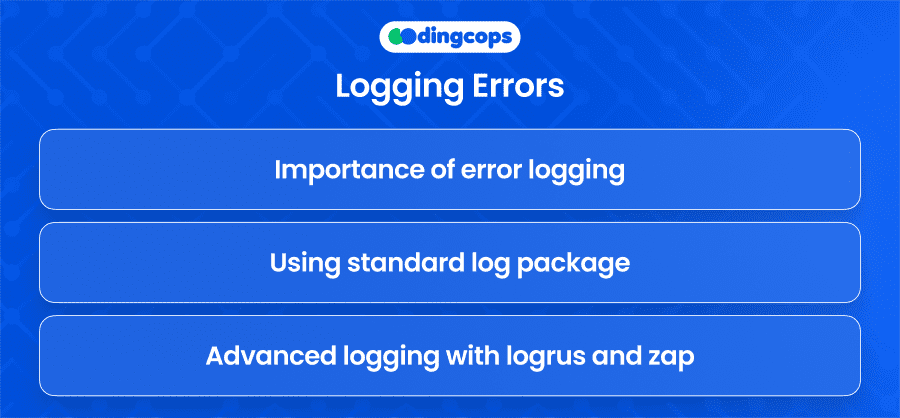
- Importance of Error Logging
Logging errors is important for maintaining a reliable system. Logs provide a historical record of what went wrong, which is invaluable for troubleshooting and fixing issues.
- Using the Standard Log Package
Go’s standard ‘log’ package provides basic logging capabilities, suitable for many applications. However, for more complex needs, third-party libraries offer enhanced functionality.
- Advanced Logging with Logrus and Zap
‘Logrus’ and ‘Zap’ are popular Go logging libraries that provide structured and high-performance logging solutions. These are particularly useful in production environments where performance and log management become critical.
Concurrent Programming
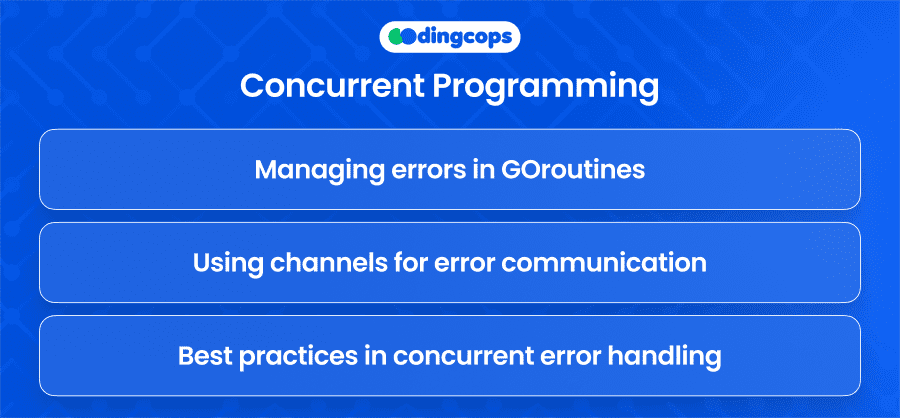
- Managing Errors in Goroutines
Error handling in goroutines requires careful consideration because goroutines may run concurrently. Using channels to communicate errors back to the main goroutine is a safe and effective approach.
- Using Channels for Error Communication
Pass an error channel as part of your goroutine’s parameters to ensure that errors are communicated back to a central place for handling.
- Best Practices in Concurrent Error Handling
Ensure that all paths that can generate an error send their errors through the designated channel and that these errors are handled appropriately.
HTTP and Web Application Errors
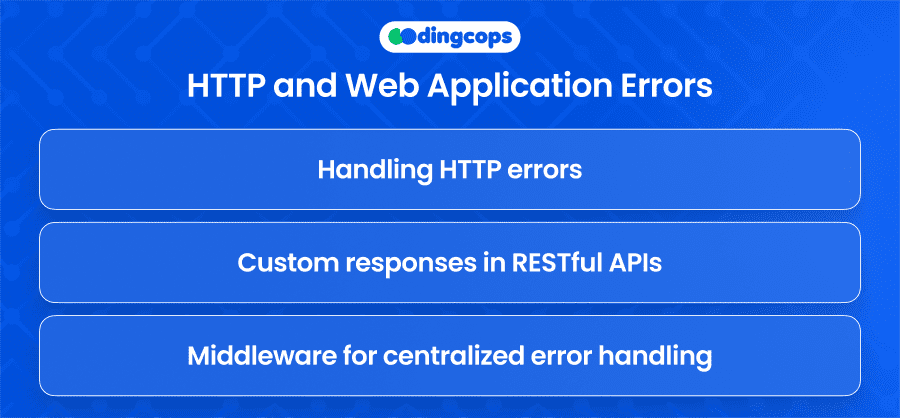
- Handling HTTP Errors
When dealing with HTTP requests, it’s important to send the correct status codes and messages to the client to indicate errors effectively.
- Custom Responses in RESTful APIs
For APIs, use structured error messages in JSON format. This helps clients handle errors predictably and is essential for good API design.
- Middleware for Centralized Error Handling
Use middleware in web frameworks to handle errors centrally rather than sprinkling error handling all over your application code.
Panic and Recover
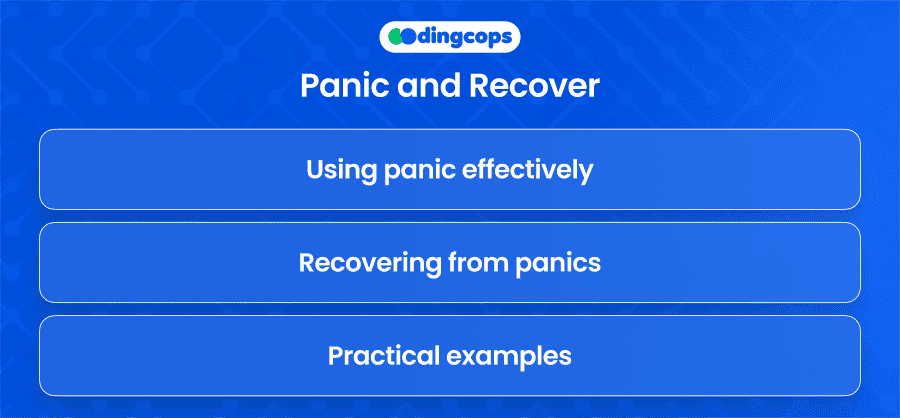
- Using Panic Effectively
Only use ‘panic’ for situations that you don’t expect to recover from in the normal flow of your program. This might include irrecoverable state inconsistencies or configuration errors at startup.
- Recovering from Panics
‘recover’ is a built-in function that regains control of a panicking goroutine. Carefully use ‘recover’ as a way to stop the panic and resume normal operations, if possible.
- Practical Examples
Showing examples of using ‘panic’ and ‘recover’ in a controlled scenario can demonstrate their proper use in Go programs.
Advanced Techniques
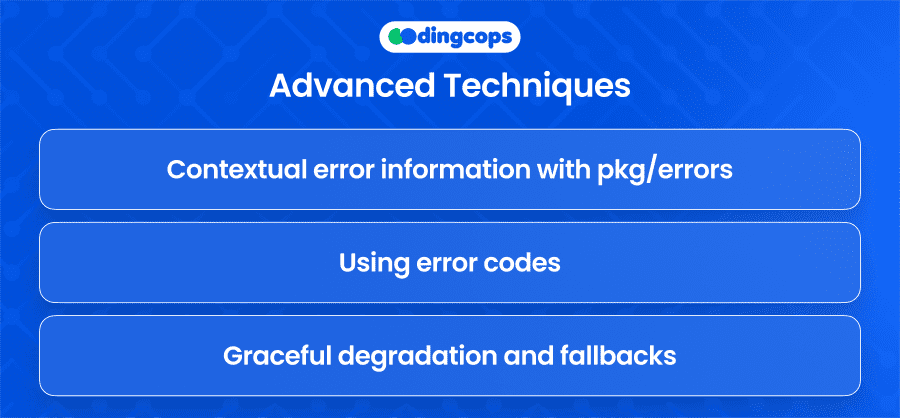
- Contextual Error Information with pkg/errors
The ‘pkg/errors’ package allows adding stack traces and other contextual information to your errors, which can be incredibly useful for debugging.
- Using Error Codes
Assigning specific error codes to different types of errors can facilitate error handling, especially when dealing with external systems like databases or networking services.
- Graceful Degradation and Fallbacks
Implementing graceful degradation or fallback logic in your error handling can improve the resilience of your application. Instead of failing outright, provide alternative solutions or minimal service continuity.
Conclusion
Effective error handling is more art than science. It requires understanding the tools and techniques at your disposal and integrating them thoughtfully into your codebase. By embracing Go’s pragmatic approach to error handling, you can write more dependable and maintainable software.