If you want to keep your application’s configuration secure and flexible, then it is essential to create and manage environment variables in ReactJS. This blog will make it easier and more engaging for the developers to use environment variables in React projects
Defining Environment Variables
When it comes to application development, environment variables play an important role. Using environment variables, developers can manage configuration values separately from the code. By this, the application becomes adaptable to different environments without any change in source code.
When you hire ReactJS developers from CodingCops, it is particularly important in ReactJS apps requirements to handle server URLs, API keys, or behavior flags separately across testing, development, and production environments.
Types of ReactJS Environment Variables
There are two main types of React environment variables:
- System-Defined Environment Variables
- User-Defined Environment Variables
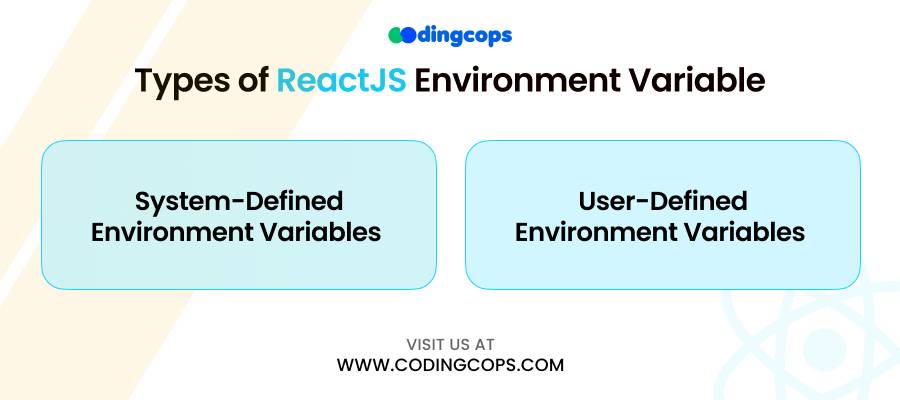
System-Defined Environment Variables
System-defined environment variables are already set into your system. For example, HOME or PATH are the system-defined environment variables that guide you toward the system path and then the home directory. These can be considered as the default settings of the system and they guide apps on where to look for different things.
User-Defined Environment Variables
As the name suggests these variables are defined by the user. User-defined environment variables can be anything from the API key to the app’s port number. They work just like your personal Pots-it notes and remind your app of different values.
Why Use Environment Variables?
There are many reasons that make using ReactJS environment variables important to use:
- Security
React environment variables keep sensitive data like API keys out of your codebase.
- Flexibility
They easily change settings between development, testing, and production without any code changes.
- Maintenance
Simplify configuration management and codebase maintenance.
Setting Up Environment Variables in ReactJS
ReactJS projects that are created with Create React App (CRA) come with built-in support for environment variables. Following is the process to set them up:
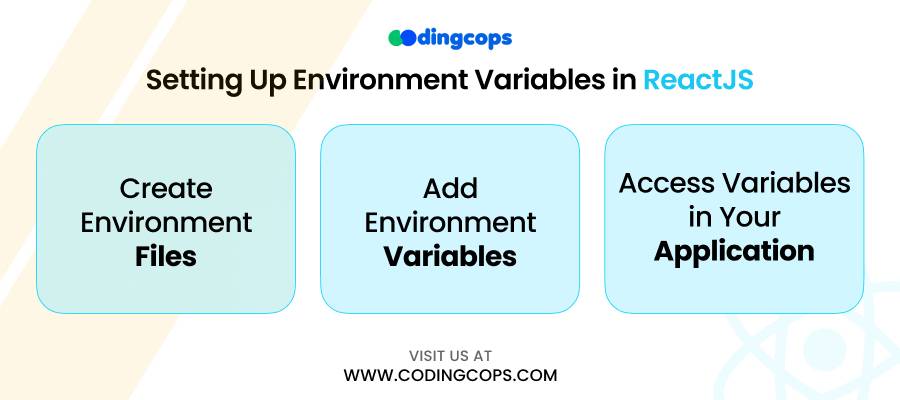
Step 1: Create Environment Files
For a standard setup, there are two main environment files:
- .env.development for development-specific settings
- .env.production for production settings
You can also add .env.local for local overrides, which are not checked into version control.
Step 2: Add Environment Variables
In your environment files, add variables starting with REACT_APP_ to make them accessible in your React app. For example:
REACT_APP_API_URL=https://api.example.com
REACT_APP_GOOGLE_MAPS_API_KEY=your_api_key_here
This prefix is mandatory for Create React App to expose these variables in process.env.
Step 3: Access Variables in Your Application
You can access these variables in your React components using process.env. For instance:
const apiUrl = process.env.REACT_APP_API_URL;
Remember, only variables that start with REACT_APP_ are embedded into the build and readable in your app.
Best Practices for Managing Environment Variables
To make the most out of ReactJS environment variables, you need to consider these best practices:
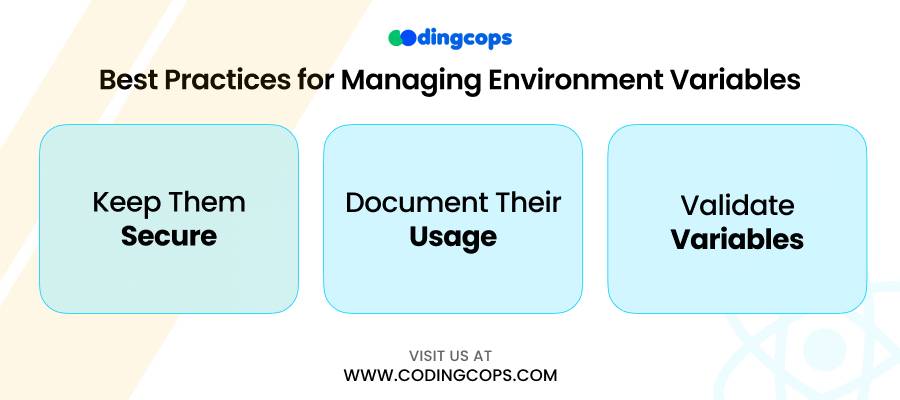
- Keep Them Secure
Do not store sensitive information directly in your repository. Use secrets management tools or add sensitive files to .gitignore.
- Document Their Usage
Ensure that your team knows what each variable is for and how to configure them properly.
- Validate Variables
Add runtime checks to ensure necessary variables are set. This prevents runtime errors due to misconfiguration.
Common Issues and Troubleshooting
Sometimes, developers encounter issues when using environment variables. Here are a few common problems and their solutions:
- Not updating in the Browser
If changes to environment variables aren’t reflecting, ensure you restart your development server after making changes to the environment files.
- Build-Time vs. Runtime
Remember that environment variables are embedded during the build process in the Create React App. Changes made after the building will not take effect until the next build.
Conclusion
React environment variables are an effective way to manage your application’s configuration accurately and securely. By understanding how to effectively set up and use these variables in your ReactJS projects, you enhance both the security and flexibility of your applications.
More Related Blogs
- React vs. Backbone.js
- Top 10 Future of React in 2024
- React Lifecycle Methods
- React State Management Libraries