React is a dominant force in modern web development because of its component-based design, efficient rendering, and management of user interface interactions. If developers wish to ace interviews, learning React interview questions might help them stand out and show off their range of skills.
This blog helps developers prepare by covering important React issues, ranging from fundamental ideas to more complex subjects. Moreover, this guide will also help companies looking to hire React developers by understanding which questions to ask.
Basic React Questions
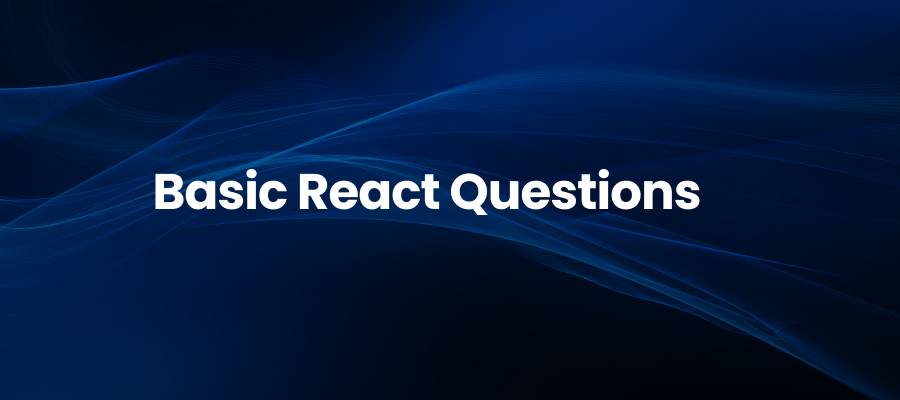
1. Why is React so popular, and what is it?
Facebook created the open-source React JavaScript framework to help developers create user interfaces, especially for single-page apps with dynamic data. It is well-liked for its effectiveness at rendering and updating the appropriate elements when data changes, which facilitates UI development.
2. Explain the concept of Virtual DOM
An abstraction of the actual DOM is the Virtual DOM. React can perform changes more quickly by producing a lightweight duplicate of the actual DOM. By comparing the Virtual DOM to the real DOM and only making required adjustments in response to changes in a component’s state, React increases efficiency.
3. What are React’s primary features?
- Component-based architecture: Components that control their own state comprise React apps.
- Declarative UI: React’s ability to update views in response to data changes simplifies the design of interactive user interfaces.
- Virtual DOM: The use of the Virtual DOM by React improves app speed.
4. How is JSX different from HTML, and what is it?
A syntactic extension called JSX (JavaScript XML) allows JavaScript to be used with HTML-like syntax. In contrast to HTML, JSX has to be compiled into JavaScript in order for the browser to understand it. It provides a way to arrange how components in JavaScript code are rendered.
5. How does React handle state and props?
Component-specific data that is subject to change over time is stored in an object called a state. Read-only information sent from a parent component to a child component is called a prop, short for properties. Props are immutable and let data flow between components, whereas state permits changing data within the component.
6. Why is unidirectional data flow necessary, and how does React accomplish it?
React enforces unidirectional data flow by leveraging props to ensure that data moves from parent components to child components. This approach makes debugging React applications and tracking state changes easier because the data flow is predictable and structured.
7. What is React’s primary difference from other JavaScript frameworks like Angular or Vue?
React is a library that focuses solely on developing user interface components; it is not a full framework. It allows developers flexibility by allowing them to choose other tools for state management or routing, in contrast to Angular, which provides an all-in-one solution. Another feature that distinguishes React in terms of performance improvement is its Virtual DOM.
8. Can React be used for mobile app development? Explain how.
Yes, React can be used to create mobile apps with React Native, a framework that lets programmers create cross-platform apps with JavaScript and React principles while rendering native iOS and Android components.
9. What is the purpose of the key attribute in React?
A list’s items can be uniquely identified using the key property. By providing appropriate diffing during reconciliation, it facilitates React’s ability to update and re-render the list as changes take place.
10. Why is JSX not mandatory in React?
JSX is optional and is used in createElement(). React may be used directly to generate React components without the need for JSX.The component structure is defined via createElement(), although JSX enhances developer efficiency and readability.
11. What is the significance of default props in React?
To define default values for a component’s properties, use default props. In the event that a prop is not specifically supplied, they guarantee that the component has a fallback value. Component.defaultProps is used in their definition.
Intermediate React Questions
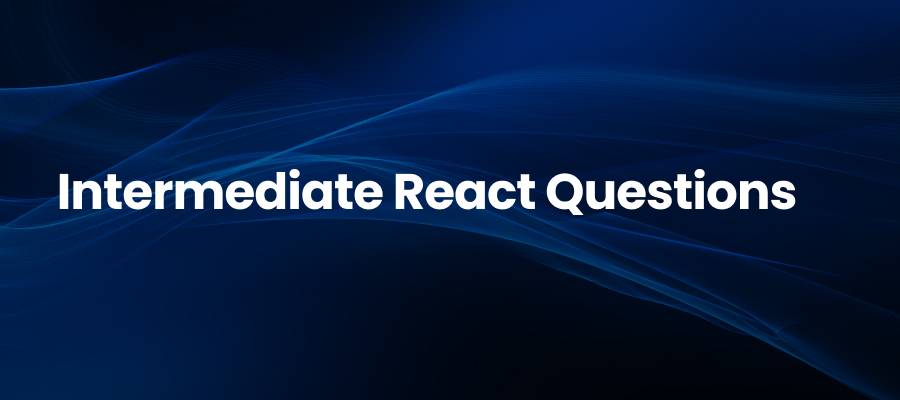
12. What is the difference between functional components and class components?
Before hooks were introduced, the typical method for building React components was to use class components. They contain lifecycle methods and extend React.Component, but functional components are more straightforward JavaScript functions that can now handle side effects and state via hooks.
13. Explain React hooks like useState and useEffect.
- useState: a hook that enables state to be added to functional elements.
- useEffect: A hook for handling side effects including data retrieval, DOM manipulation, and subscription setup. It may be set up to run in response to particular state changes and executes after the render.
14. How does conditional rendering work in React?
When certain requirements are met, components can show other elements or components thanks to React’s conditional rendering capability. This may be accomplished using JavaScript’s conditional operators, including if-else, ternary operators, and logical &&.
15. What are higher-order components?
Functions that take a component and return a new one are known as HOCs. They are used to give components additional functionality, such logging, authentication checks, and data fetching.
16. How do you manage forms in React?
React form handling entails keeping form data in state. React is used by controlled components to maintain their state; onChange handlers are used to update state in response to user input. Referees can be used to manage uncontrollable elements.
17. What are keys in React, and why are they important?
React uses keys to determine whether items in a list have been added, modified, or deleted. This preserves the identity of the component and aids in rendering efficiently. React could re-render list items needlessly if keys are absent.
18. What are fragments in React, and when would you use them?
You may group several child items together using fragments without creating an additional DOM node. When presenting lists or components that need a flat DOM structure, they are helpful in reducing superfluous wrappers. Syntax: or <>.
19. How does React handle error boundaries, and why are they useful?
Error borders are React components that, rather than crashing the application, report JavaScript failures in their child component tree and show a fallback user interface. They are helpful in keeping a malfunctioning component from impacting the overall application.
20. How do stateful and stateless components vary from one another?
Stateful components, which are usually designed as class components or functional components with hooks, are able to update dynamically and handle their own state internally. Conversely, stateless components do not internally handle state; instead, they generate user interfaces (UI) based solely on props.
21. How do portals work in React, and what are their common use cases?
Portals provide a way to render children into a DOM node outside the parent component’s DOM hierarchy. Common use cases include modals, tooltips, and dropdown menus where the rendered content should appear outside the main DOM structure for styling or z-index purposes.
22. What are React Refs, and how do you manage them?
In React, references offer a direct method of accessing a DOM element or a component instance. React is used in their creation.createRef(), and the ref property allows it to be affixed to items. Common uses for references include controlling attention, starting animations, and incorporating third-party libraries.
23. What are synthetic events in React?
React’s cross-browser wrapper for native browser events is called Synthetic Events. They add event pooling for better speed and guarantee consistent behavior across many browsers.
24. How are render props different from higher-order components (HOCs)?
HOCs are functions that take a component and provide a better version of it. They are employed to allow components to share logic. Similar objectives are accomplished using render props, which dynamically pass logic or data by utilizing a function as a child.
25. How does useMemo differ from useCallback in React?
- By memoizing a computation’s outcome, useMemo avoids costly value recalculation.
- useCallback memoizes a function, preventing unnecessary re-creations of the same function reference.
Advanced React Questions
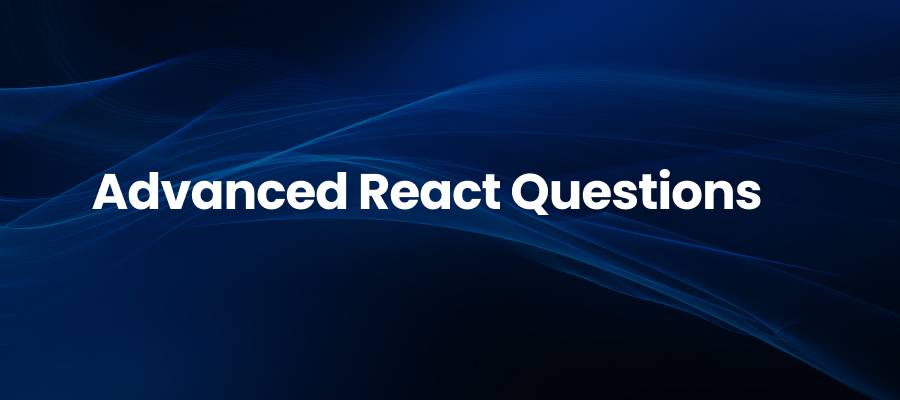
26. Explain the concept of React context and its use cases.
Data sharing across components is made possible by the React Context API, which eliminates the need for explicit prop handing at every stage. It is employed to handle global state, including theme choices and user authentication status.
27. What is the difference between Redux and the Context API?
Redux is more powerful and offers middleware for more complex tasks like asynchronous actions, yet both technologies aid in state management. For smaller applications when a comprehensive state management library is not required, the Context API is more straightforward and appropriate.
28. How does server-side rendering work in React?
SSR entails providing the client fully rendered pages after rendering React components on the server. This method is good for SEO and can speed up initial load times. SSR implementation in React is made simpler by frameworks like Next.js.
29. Explain the React lifecycle methods in class components.
Lifecycle methods in class components include:
- componentDidMount: Runs following the mounting of the component.
- componentDidUpdate: Runs subsequent to the component’s upgrade.
- componentWillUnmount: Operates prior to the component being taken out.
- shouldComponentUpdate: Decides if a component needs to be rendered again.
30. How can the performance of a React application be improved?
Optimizing React applications can involve:
- React.memo() is used to avoid needless re-renders.
- Components are loaded slowly to shorten the initial load time.
- Use dynamic imports to divide code.
- Using context or lifting state to optimize state management.
31. Describe React Fiber’s idea and how it enhances React’s functionality.
The foundational architecture of React that makes incremental rendering of the Virtual DOM possible is called React Fiber. It breaks rendering work into units and spreads them across multiple frames, improving responsiveness and handling complex updates efficiently.
32. How does code splitting using React.lazy and Suspense function in React?
You may use code splitting to break up your application into smaller bundles and speed up load times. While React.lazy() enables components to load slowly, suspense is used to display fallback content while the component loads asynchronously.
33. How can you debounce user input in React to optimize performance?
Debouncing delays the execution of a function until after a specified period of inactivity. In React, this can be implemented using setTimeout and clearTimeout in an onChange handler. Libraries like Lodash also provide debounce functions.
34. What are render props, and how do they differ from higher-order components?
Render props are a pattern in which a component determines what to render by using a function prop. Render props reveal a technique for creating dynamic content, as opposed to HOCs, which cover components to offer improved functionality.
35. How do you handle memory leaks in React applications?
Memory leaks can be handled by:
- Cleaning up effects in useEffect with a return function.
- Avoiding setting state in unmounted components.
- Clearing intervals, timeouts, and event listeners when they are no longer needed.
36. What is the purpose of React Context, and how do you use it?
Without having to manually transmit props across each level, React Context offers a means to communicate data (such as themes or authentication) among components. React is used in its creation.createdContext() and used by the hooks for Provider and useContext.
37. What is React’s Concurrent Mode, and what are its benefits?
Concurrent Mode is a collection of characteristics that enable React to pause rendering operations, enhancing the responsiveness of apps. Benefits include support for elements like suspense, improved user experience, and more seamless UI upgrades.
38. What are React hooks rules, and why are they important?
- Only call hooks at the top level of a function.
- Only call hooks in React functional components or custom hooks.
These rules ensure the correct ordering of hooks and maintain predictable behavior during re-renders.
React Ecosystem Questions
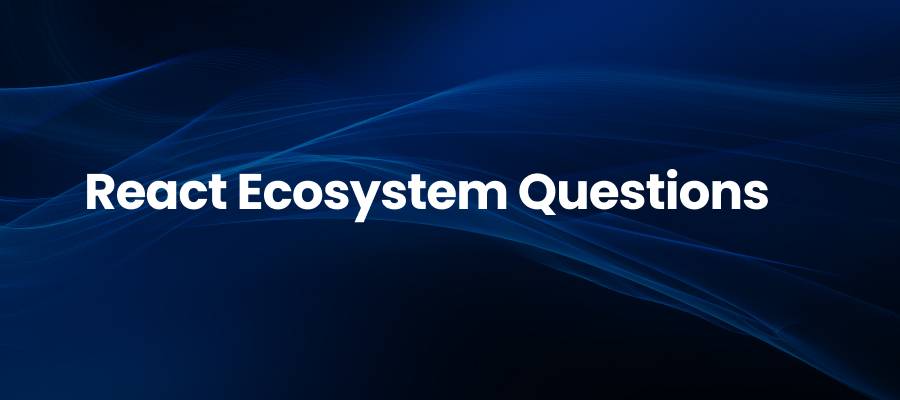
39. How is a React router utilized, and what is it?
React Router is a popular library for routing in React. It enables switching between different components without requiring a page reload. Developers use the,, and components to set up routing.
40. Describe how Redux is used in state management.
JavaScript apps utilize a package called Redux to control global state. The application state is predictable and easier to debug due to its one-way data flow. It uses actions, reducers, and a store to manage state.
41. What distinguishes Redux Saga from Redux Thunk?
Tools for middleware Asynchronous Redux operations are managed via Redux Thunk and Redux Saga. While Saga employs generators to manage more intricate asynchronous processes and side effects, Thunk enables functions to be dispatched.
42. How does React Query handle data fetching?
State management and data retrieval are made easier using React Query. Apps become more responsive and optimized as a result of its automated caching, synchronization, and refetching of data as needed.
43. What are popular tools for testing React applications?
- Jest: A testing framework for JavaScript.
- Enzyme: A tool to render components and make assertions.
- React Testing Library: Encourages testing user interactions rather than implementation.
44. How do you implement PropTypes in React, and what is their purpose?
PropTypes validate the types of props provided to a component, ensuring that the correct data types are used. To implement them, import PropTypes and use Component.propTypes to define the required types.
45. How does Recoil compare to Redux for state management?
Compared to Redux, Recoil is easier to use and lighter, and it has a built-in method for handling both local and global state. It enables fine-grained updates and works in unison with React. For larger, more intricate apps requiring sophisticated middleware, Redux is more potent.
46. Describe the function of Babel and Webpack in a React application.
JavaScript scripts and other components are compiled into a single deployment bundle by Webpack, a module bundler. A JavaScript transpiler called Babel transforms JSX and contemporary JavaScript (ES6+) into browser-compatible JavaScript. When coupled, they enable modern React development methodologies.
47. How can React applications be made to include micro-frontends, and what are they?
An architectural technique known as micro-frontends combines several small, independent frontend programs into a single web application. React supports this by allowing separate teams to develop components or sections independently, integrating them at runtime via module federation or other tools.
48. How does React Router function and what is its role?
Client-side routing is managed by React Router. It allows you to render components based on the URL. It works by matching the current URL with a Route and rendering the associated component.
49. How does React Router function and what is its role?
Client-side routing is managed by React Router. It allows you to render components based on the URL. It works by matching the current URL with a Route and rendering the associated component.
50. What state management differences exist between Redux and Context API?
Redux is a state management system with devtools, immutability, and middleware among its features. The lightweight state sharing strategy of the Context API is perfect for small to medium-sized applications.
51. How do styled-components function in React, and what are they?
A library called Styled-components is used to write CSS in JS. It allows you to define styles within components as JavaScript objects, leveraging template literals to generate class names dynamically.
Common Coding Challenges in React Interviews
- Implementing a counter app
- Creating a to-do list
- Demonstrating conditional rendering
- Fetching data from an API
- Building custom hooks
52. In a React application, how would endless scrolling be implemented?
Implementing infinite scrolling involves determining the scroll position, listening for the scroll event, and retrieving further information when the user approaches a threshold close to the bottom of the page. Libraries like react-infinite-scroll-components simplify this process.
53. Create a function in React that debounces a search input field.
import React, { useState } from ‘react’;
import _ from ‘lodash’;
function SearchInput() {
const [query, setQuery] = useState(”);
const handleSearch = _.debounce((input) => {
console.log(‘Searching for:’, input);
}, 300);
const handleChange = (e) => {
const value = e.target.value;
setQuery(value);
handleSearch(value);
};
return <input type=”text” value={query} onChange={handleChange} />;
}
54. Build a component that fetches and displays paginated data from an API.
import React, { useState, useEffect } from ‘react’;
function PaginatedList() {
const [data, setData] = useState([]);
const [page, setPage] = useState(1);
useEffect(() => {
fetch(`https://api.example.com/data?page=${page}`)
.then((res) => res.json())
.then((newData) => setData((prev) => […prev, …newData]));
}, [page]);
const loadMore = () => setPage(page + 1);
return (
<div>
<ul>
{data.map((item) => (
<li key={item.id}>{item.name}</li>
))}
</ul>
<button onClick={loadMore}>Load More</button>
</div>
);
}
Behavioral and Conceptual Questions
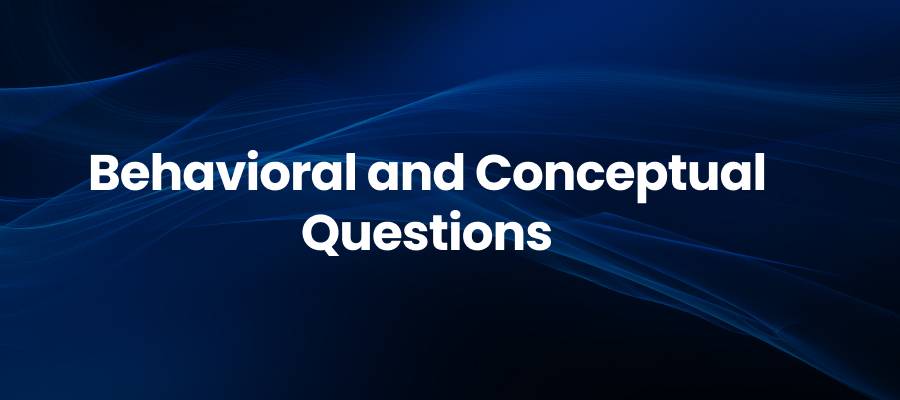
55. How do you stay updated with the latest changes in React?
Following official React documentation, subscribing to popular React blogs, joining React communities, and attending relevant webinars or conferences.
56. Share a challenging bug you resolved in a React project.
Problem-solving talents are sought after by employers, thus explaining a difficult bug and how you resolved it shows your analytical prowess.
57. Give an example of a time you utilized React to implement a sophisticated feature.
Highlighting the implementation of intricate features such as dynamic forms, nested routing, or data visualization helps interviewers see your practical experience.
58. How do you ensure scalability in a React application?
Proper component structure, effective state management, and modular code are essential for building scalable React applications.
Bonus: Quick Tips for Acing React Interviews
- Understand React’s core principles deeply.
- Gain hands-on experience through building real-world projects.
- Practice explaining your code clearly.
- Stay current with the newest features and industry best practices.
Conclusion
A developer’s confidence and preparedness may be greatly increased by mastering these 50+ React interview questions. React interviews often go beyond theoretical knowledge, so hands-on practice is crucial. Take time to explore these topics, refine your understanding, and you’ll be well-prepared for any React interview.