The horizon of software development is vast, and so are the frameworks and languages that assist in development. Today, CodingCops has come up with 50+ Node.js interview questions that are considered the essence of Node.js for interview purposes.
This guide aims to help beginners and professionals to prepare themselves for the Node.js interview. Moreover, business owners and professionals looking to recruit Node.js developers for their applications can also benefit from this.
Beginner-Level Node.js Questions
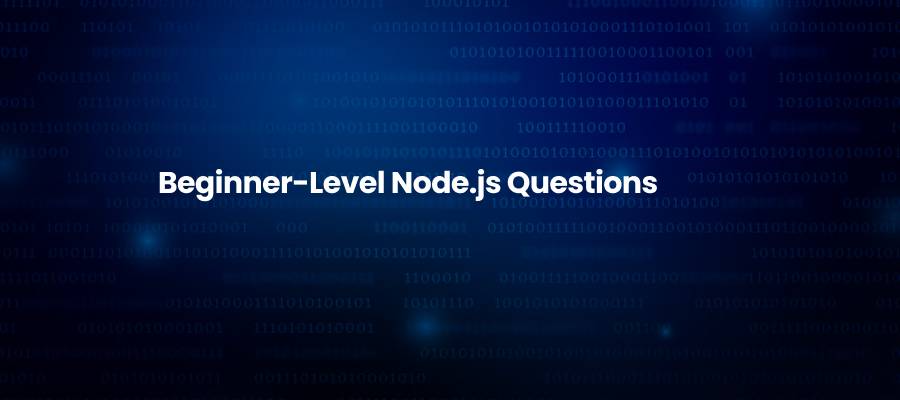
- What does Node.js mean and why it is used?
Node.js is an open-source server-side runtime environment created on the Javascript platform that focuses on developing high, optimized, and scalable network applications. They are used for applications that require real-time interaction, such as chat apps, APIs, and web servers because of non-blocking and event-driven features.
- Describe characteristics of Node.js.
Non-blocking I/O operations, event-driven architecture, and high responsiveness are the core characteristics of Node.js. It is capable of handling multiple requests concurrently. It gives many packages via npm which assist in building small and fast applications.
- Differentiate between Node.js and JavaScript.
Node is a system that makes it possible for JavaScript to run on servers, whereas JavaScript is a programming language that primarily functions in browsers. A collection of JavaScript modules and libraries called Node.js is used for server-side programming.
- What is the roll of NPM in Node.js?
NPM is used to install, manage, and distribute packages for Node.js applications also known as Node Package Manager. It eliminates the redundancy of managing dependencies and is crucial for today’s JS & Node.JS application development.
- Describe a little about the event-driven architecture of Node.js.
Node.js performs tasks utilizing an event loop that waits for an event and performs a callback function. This design makes it very efficient that it can work on many requests at the same time waiting for each operation to be done.
Core Concepts Questions
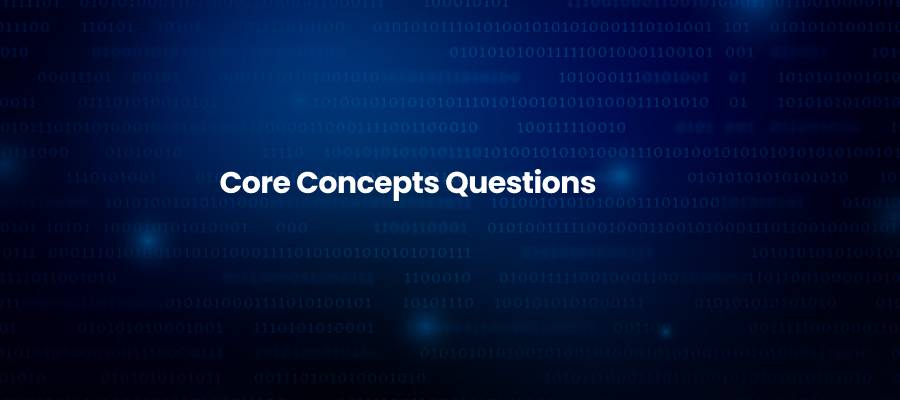
- What does a non-blocking I/O mean in Node.js?
Nonblocking I/O means the Node.js does not need to wait for the prior tasks to complete to perform multiple input/output operations. This feature enhances the operation and elasticity of an application, particularly in real-time applications.
- What is require() function?
The require() function in Node.js helps to use modules or files in an application to avoid the repetition of similar codes. It is a useful means of keeping the large projects modular and well-structured, especially from the perspective of their author.
- Describe the role of exports in Node.
The exports object is used to define the parts of a module that can be accessed by other files. By exporting specific functions or objects, you can create reusable and modular code components.
- How do you manage asynchronous operations?
Concurrency in Node is done using callbacks, Promises, or async/await in Node.js. Such approaches enable the application to perform other operations concurrently when using facilities such as an API call or reading a file.
- What is the event loop?
The event loop is one of the main features of Node.js. It is designed for handling asynchronous operations. It waits for events and performs the related callback functions, the mechanisms of asynchronous I/O, and makes concurrent jobs more effective.
Modules and Packages Questions
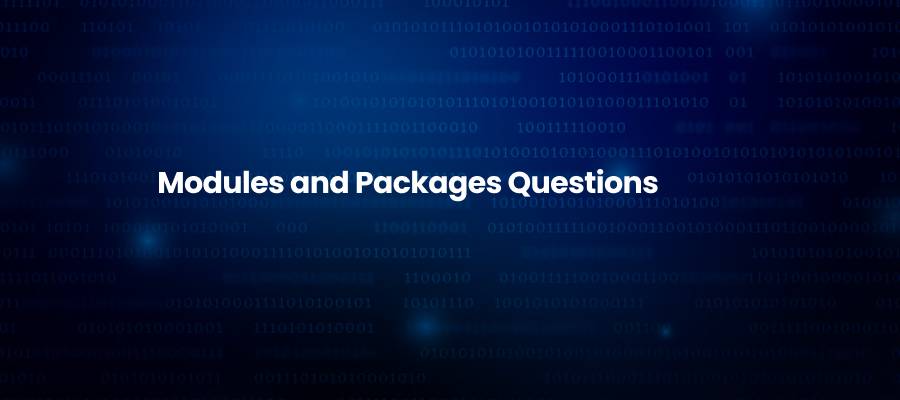
- What are Node.js modules?
A module in Node.js is an individual file or package that contains some of the repeated pieces of code. HTTP and fs are standard modules that come with Node.js while other modules, can be developed to contain particular functionalities.
- What do you understand by local and global modules?
Local modules are used only within a project and are installed in a directory specific to that project. Whereas, global modules are installed throughout the system and are accessible from any Node.js application.
- How to develop your own module?
To generate a module, one has to write the necessary functionality in a .js file, and then use the module.exports command. Then you have to use the required function to bring that module into other files you are working on.
- What is package.json?
package.json is file in Node.js where the project name and version, dependencies, or scripts can be located. It becomes relevant in terms of the configuration of the project and the development environments of the project too.
- What is the difference between dependencies and devDependencies?
Dependendencies are needed for the application to run in production and devDependencies are all the tools and frameworks used during development, for example testing or building.
File Systems and Streams Questions
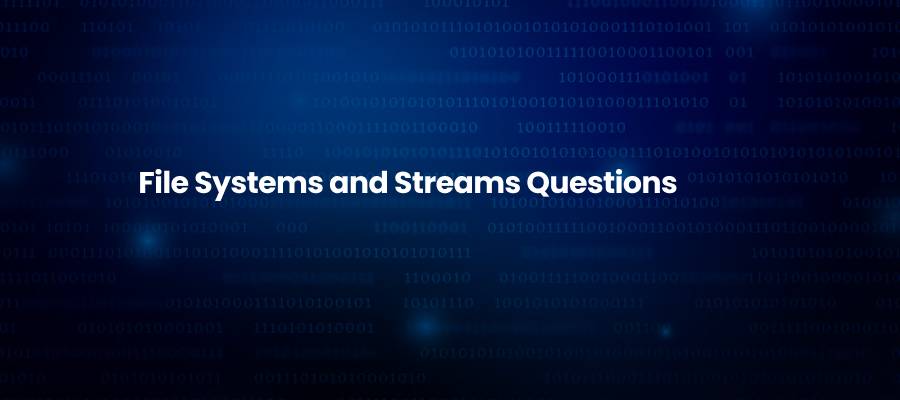
- What are streams in Node.js?
Streams are used for processing data that is read or written a block at a time. It is especially important for working with big files or streaming data through a network while being very frugal with memory.
- How does the fs module work?
The fs module is the interface to file system operations such as read and write, and creation and deletion of files. It provides both synchronous and asynchronous modes for the operation of file activities.
- What is fs.readFile and fs.createReadStream?
The fs.readFile reads the whole file at a time and this takes more time when working on big files. fs.createReadStream reads the file in chunks and hence one can work on big chunks of data without exhausting the system.
- Describe and compare synchronous file reading and asynchronous file reading.
Synchronous file reading freezes the running program until the operation concerning the file is finished. In contrast, Asynchronous file reading or writing enables the program to perform other functions while the file operation is still on.
- What do we mean by a writable stream and a readable stream?
Readable streams are used if the data is to be read from a source while writable streams is used when data need to be written to a destination.
APIs and HTTP Questions
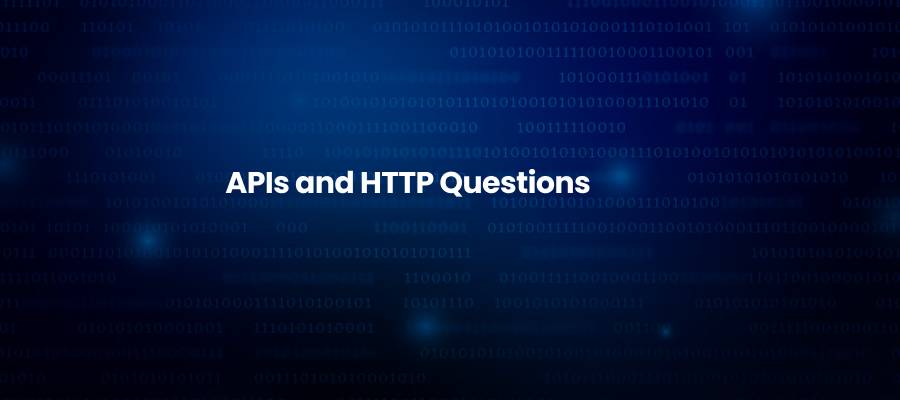
- How to create an HTTP server?
For creating an HTTP server in Node.js, use the http module and define a server with the createServer method.
Here is an example:
const http = require(‘http’);
const server = http.createServer((req, res) => {
res.write(‘Hello, World!’);
res.end();
});
server.listen(3000);=
- What are the key components of HTTP requests and responses?
HTTP requests include the method (GET, POST), URL, headers, and body. Responses consist of a status code (success in this case 200), a list of headers, and an optional message body that holds the requested information.
- What steps do you take when there is an error in the HTTP server?
In an HTTP server, it is possible to handle errors within middleware or using try…catch statements to catch and handle the errors easily.
- Are HTTP and HTTPS the same?
No, they’re not similar. While the HTTPS module offers a secure connection via SSL/TLS encryption, the HTTP module is for regular HTTP communication.
- What is meant by middleware in Node.js?
Middleware functions in Node.js act upon system requests and responses before they get to the terminal handler. Some of these are authentication, logging, and request parsing.
Experienced-Level Node.js Questions and Answers
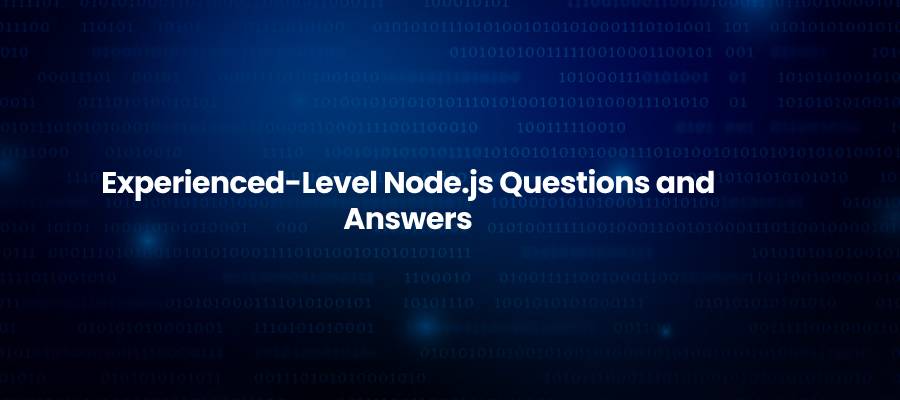
Advanced Concepts
- What is the purpose of the cluster module?
The cluster module allows Node.js to utilize multiple CPU cores by creating child processes that share the same server port. This improves performance and scalability for applications handling heavy loads.
- Explain middleware chaining in Node.js.
Middleware chaining refers to passing control from one middleware function to the next using the next() function. This approach creates a pipeline for request processing, enabling modular handling of logic like authentication or error handling.
- What are worker threads in Node.js and when can they be utilized?
The worker threads are useful for running JavaScript code outside of the event loop and in parallel threads. They are perfect for CPU-bound operations that would otherwise freeze the main thread such as data processing or image manipulation.
- How does memory management work at Node.js?
Node.js leans back on V8’s garbage collector. It takes care of memory management by disposing of objects that are no longer in use. However, the developer needs to be careful not to leave memory leaks by observing the objects actively and releasing pointers to the unwanted variables.
- What is a process and thread in Node.js?
The process is an instance of a program in execution that has its own memory space, whereas threads are lighter-weight processes that have their program counters but share the same memory space. As a fact, acting single-threaded by default, Node.js can start child processes or worker threads if needed.
- Differentiate between setImmeidate() and process.nextTick()?
process.nextTick() reports callbacks to be executed after the current operation and before any I/O events while setImmediate() reports callbacks to be run in the next cycle of the event loop.
- How can you optimize your Node.js application?
Caching frequently occurring data, employing clustering to leverage multiple cores, effectively managing database queries, and utilizing tools such as PM2 for application monitoring and scaling are all examples of performance optimization.
- What kind of issues are frequently found to cause poor performance in Node.js applications?
Some of the performance concerns that may be experienced are due to block operations, non-optimized databases, numerous middleware, or poor execution of large datasets. Such bottlenecks can be easily spotted through profiling and subsequent monitoring processes.
- Describe how caching can be done in a Node.js application.
Caching can be done with the help of in-memory systems such as Redis or Memcached. Cache helps applications minimize frequent queries to the database and in the process helps increase application response time.
- What is a memory leak? How to prevent memory leaks?
When memory that is no longer required is not released, it can cause a memory leak, which eventually results in excessive memory usage. Avoid global variables, close resources correctly, and use tools like heapdump to keep an eye on memory usage to avoid it.
Testing and Debugging
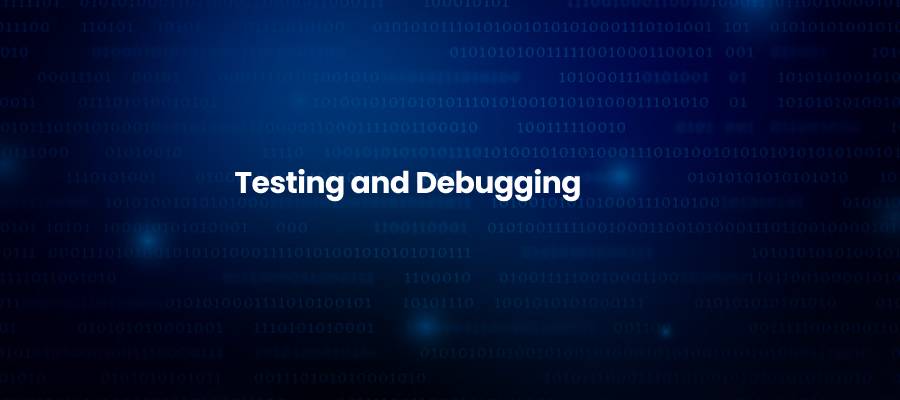
- How to debug a Node.js application?
You can debug the Node.js applications by using the built-in debugger, and console.leg statements. Moreover, one can utilize external tools like Chrome DevTools, etc, for debugging.
- Describe how you can use Mocha or Jest while testing in Node.js.
Mocha is a testing tool that provides structure to tests for developers who write them out. Jest is a testing framework used for everything from mocking to snapshot testing. Both help run Node.js applications and also help in testing applications.
- What is the role of the assert module in Node.js?
The assert module is used for simple checks of situations using conditions, i.e. it is used for assertation. That is most helpful when you have function tests or when you are expecting something in the outcome of a function.
- What is your strategy for handling promise injections in Node.js?
Unhandled promise rejections can be managed using .catch() for promises or by listening to the process.on(‘unhandledRejection’) event. This ensures proper logging and recovery from unexpected errors.
- Tell the names of some common tools used for testing Node.js applications.
For testing Node.js applications, one can rely on tools like Mocha, and Jest.
Security
- How do you secure an Express.js application?
Securing an Express.js application involves using libraries like Helmet.js for setting HTTP headers, validating user input, enabling HTTPS, and implementing authentication mechanisms like OAuth or JWT.
- Describe a few common Node.js application vulnerabilities.
Typical Node.js application vulnerabilities include SQL injection, CSRF, and cross-site scripting.
- Why Helmet.js is used?
By including HTTP headers that guard against attacks like clickjacking, cross-site scripting, and content sniffing, Helmet.js improves security. It’s a straightforward but efficient method of enhancing application security.
- Can you prevent SQL injection in Node.js application?
SQL injection can be prevented in Node.js applications by using prepared statements, parameterised queries or ORM frameworks such as Sequelize.
- How to protect data?
Access sensitive data by encrypting it with libraries like crypto, using environment variables for credentials, and implementing authentication and authorization to such data.
Database Integration
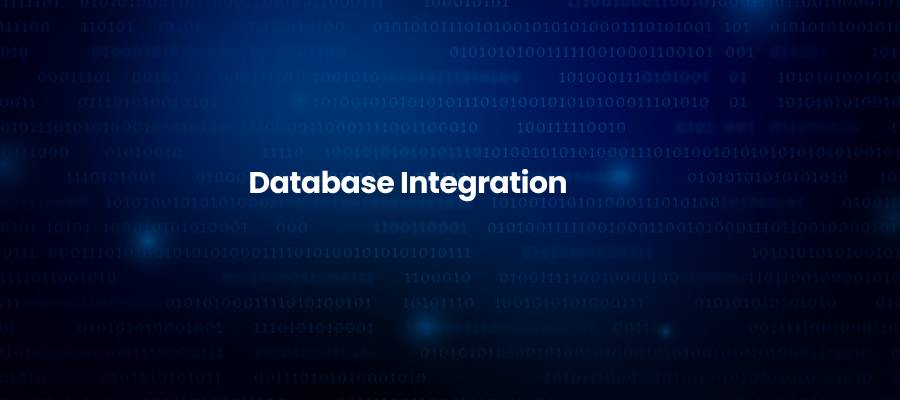
- Explain the finest way to connect a Node.js application with database?
Make use of an ORM library or database driver tailored to your database (e.g., Sequelize for SQL, Mongoose for MongoDB). To ensure secure communication, specify the connection string and set up authentication.
- What is the main difference between Sequelize and Mongoose?
Sequelize is an ORM for SQL databases that allows to work with tables as objects of JavaScript. Mongoose being an ODM for MongoDB allows a user to interact with the documents within NoSQL databases.
- What strategies do you use to handle database queries in Node.js?
Promises or async/await can be used to handle database queries to guarantee non-blocking execution. Reliable interactions depend on proper validation and error handling.
- What is a connection pool?
It is a cache of database connections that is reused to manage several requests efficiently. Using a connection pool reduces the overhead of creating and closing connections for every connection.
- What is the use of ORMs in Node.js?
Developers can work with objects rather than raw SQL thanks to Object-Relational Mapping (ORM) libraries like Sequelize, which abstract database operations. Code becomes easier to read and maintain as a result.
- Tell me the best way to deploy a Node.js application in production.
Use platforms like AWS, Heroku, or Docker for deploying your Node.js application.
Rounding Off
To become proficient with Node.js, one must grasp its foundations and explore its more complex features. This thorough guide provides a solid basis for overcoming obstacles and succeeding as a developer by answering important interview questions. Learn these tips, get some coding experience, and hone your abilities to succeed in any Node.js position!